The information age is over: we have all the information now. All of it. We're in a unique place in human history where we've somehow managed to mine more data than we know what to do with... and a lot of that data is easily accessible via APIs.
We're going to get our feet wet with REST APIs today, thus allowing us to interact with meaningful information. Making Ajax GET calls with JQuery is perhaps as basic as it gets: there's a good chance we already know all this stuff, but even I constantly forget the exact syntax of everyday functions. Chances are I'm going to come back to this post at some point to copy and paste the snippets below.
Introduction to REST APIs
If you're new to executing AJAX requests, you may be new to REST APIs. This crash course will be fast and rough around the edges, so strap in.
In the most simple sense, a REST API endpoint is a URL. It just so happens that this URL probably expects more from you than simply visiting it, and as a result, should output something useful for you. API Endpoints will almost always output either JSON or XML; these responses will give you information varying from error codes to the data you seek.
REST APIs expect requests to come in one of the following forms:
- GET: A request looking for read-only data. Some GET requests need to be copied and pasted into a browser window to receive results, but usually, we need to either authenticate or specify what we're looking for.
- POST: A write request to the target resource. Expects that new information will come as a result of this request.
- PUT: Updates pre-existing data somewhere, likely in some database.
- PATCH: Somewhat similar to PUT, and in my experience rarely used at all.
- DELETE: Expects that information will be deleted as a result of the request
If this all seems like new information, I'd highly recommend downloading Postman to become familiar with how API calls are structured.
For now, we'll focus on working with a simple GET endpoint.
Learning By Doing
To demonstrate how to make API calls via a frontend client with JQuery, we'll be walking through how to create link previews using the LinkPreview API. This service serves as a good tutorial because:
- It's an example of a simple GET endpoint
- There's a quick and immediately useful end result
- It's free
Tell me That Ain't Insecurr
I want to stress here that we're doing this for the sake of learning; while this is probably the fastest way to start working with an API, it is most definitely not secure.
Making calls with private keys stored and passed via the client side exposes your key publicly. In a production environment, this is like shoving your password in people's faces. People will most definitely want to steal and exploit your private key: if what you were doing didn't have any value, it wouldn't require a key in the first place.
Hopefully, this has scared you enough to consider passing credentials. That said, there's another solid reason we selected LinkPreview as today's example. LinkPreview offers domain whitelisting for requests, so even if somebody did steal your key, they'd only be able to use it from your domain ;).
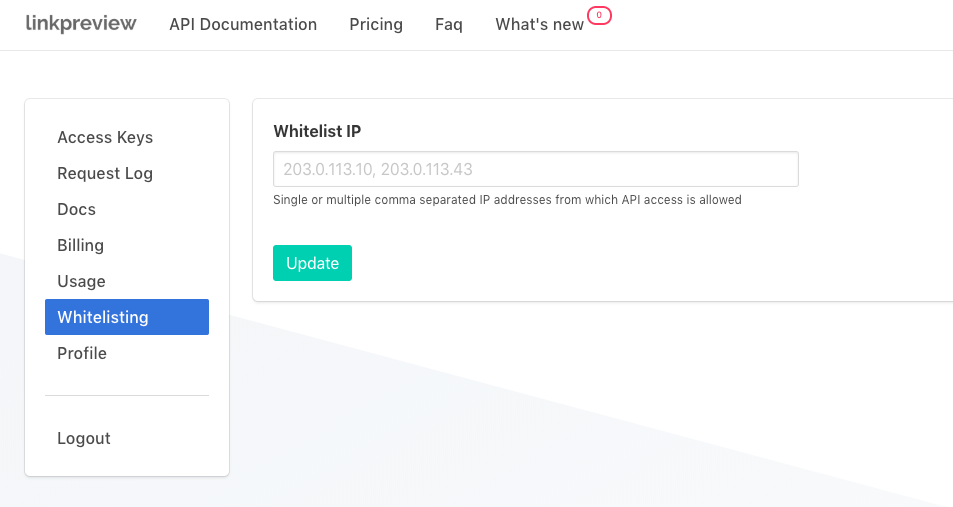
Fetch Me Daddy
Get started with an API key over at LinkPreview if you're following along. I assume you already have JQuery readily available from here forward.
To get started, we'll wait for our document to load, and set two critical variables: the API URL, and our API key.
$( document ).ready(function() {
let endpoint = 'https://api.linkpreview.net'
let apiKey = '5b578yg9yvi8sogirbvegoiufg9v9g579gviuiub8'
});
Set API endpoint and key
If you're following along with what we've done with Lynx Roundups, our next step is to get all the relevant <a>
tags on a page, loop through them, and replace them with their respective link previews:
$( document ).ready(function() {
let endpoint = 'https://api.linkpreview.net'
let apiKey = '5b578yg9yvi8sogirbvegoiufg9v9g579gviuiub8'
$( ".content a" ).each(function( index, element ) {
console.log($( this ).text());
}
});
Loop over <a>
tags
JQuery's .each
method creates a loop that iterates over every element matching the provided selector. In our example, we only want <a>
tags in the content of our page; otherwise, we would get all links, like navigation links and so forth.
Now it's time to bring in that $.ajax()
thing we've been going off about.
$( document ).ready(function() {
let endpoint = 'https://api.linkpreview.net'
let apiKey = '5b578yg9yvi8sogirbvegoiufg9v9g579gviuiub8'
$( ".content a" ).each(function( index, element ) {
$.ajax({
url: endpoint + "?key=" + apiKey + " &q=" + $( this ).text(),
contentType: "application/json",
dataType: 'json',
success: function(result){
console.log(result);
}
})
});
});
HTTP request with JQuery AJAX
This is how Ajax requests are structured: the contents of $.ajax()
is essentially an object taking values it will use to construct the request. The above example is about as simple as it gets for making a barebones GET call. We're looping through each <a>
tag and passing its contents (the URL) to the API, and receiving an object in response.
Ajax requests can take way more parameters than the ones we just specified. I recommend reading over the JQuery Ajax documentation closely, not only for the sake of these requests but understanding the potential items we can specify will solidify an understanding of REST APIs in general.
The line contentType: "application/json"
specifies that the content coming back to us will be in JSON format - this is a very common header when dealing with REST APIs.
With any luck, your response should come back looking like this:
{
"title":"Google",
"description":"Search webpages, images, videos and more.",
"image":"https//:www.google.com/images/logo.png",
"url":"https://www.google.com/"
}
JSON response from our AJAX request
If you'd like to use this in a meaningful way, feel free to do something like the mess I've put together below:
$( document ).ready(function() {
let endpoint = 'https://api.linkpreview.net'
let apiKey = '5b578yg9yvi8sogirbvegoiufg9v9g579gviuiub8'
$( ".content a" ).each(function( index, element ) {
$.ajax({
url: endpoint + "?key=" + apiKey + " &q=" + $( this ).text(),
contentType: "application/json",
dataType: 'json',
success: function(result){
$( element ).after(
'<a href="' + result.url + '"> \n ' +
'<div class="link-preview"> \n ' +
'<div class="preview-image" style="background-image:url(' + result.image + ');"></div> \n ' +
'<div style="width:70%;" class="link-info"> \n ' +
'<h4>' + result.title +'</h4> \n ' +
'<p>' + result.description +'</p> \n ' +
'</div><br> \n ' +
'<a href="' + result.url + '" class="url-info"><i class="far fa-link"></i>' + result.url + '</a> \n ' +
'</div></a>');
$( element ).remove();
}
})
});
});
Generate HTML embed from JSON response
That template should serve you well for most GET API calls you will make via JQuery. Go wild and see what you can do to leverage APIs and expose some people's personal data or whatever.
If we were to truly complete this example, we'd want to refine our logic to ensure we're not receiving nonsense. There's no validation on what's coming back in these calls, so there's nothing in place to protect us if a page doesn't comply with our format.